cURL is a tool for transferring data between servers, which makes it useful for web scraping. Sometimes, websites block cURL requests because they use anti-scraping measures. Luckily, you can bypass these measures by using proxies alongside your cURL requests.
This guide explains how to use cURL with proxies to ethically circumvent anti-cURL scraping protections.
What is cURL?
cURL is a command-line tool and library that transfers data between a client and a server over a variety of protocols.
Its flexibility, reliability, and cross-platform support make it useful for working with APIs, data scraping, or automated tasks.
cURL (Client URL) transfers data using protocols such as HTTP, HTTPS, FTP, and SMTP. It lets you customize requests by setting headers, cookies, query parameters, and HTTP methods (GET, POST, PUT, DELETE). cURL also supports error handling and debugging, which makes it useful for API testing, data retrieval, and troubleshooting network issues.
When you use proxies with cURL, you can bypass restrictions, hide your IP address, and avoid IP bans.
Why use cURL with proxies?
Proxies act as intermediaries between cURL and the target server by routing requests through different IP addresses or geographic locations.
When you use proxies, cURL gains additional capabilities, including the ability to bypass geographic restrictions, hide your real IP address, and handle many requests without triggering bans.
Bypass geo-restrictions
Geo-restrictions limit access to some website content and online services based on location. By using a proxy server located in a specific region, cURL can reroute your requests to appear as though they originate from that region. This allows you to access localized versions of websites, regional APIs, or otherwise restricted content.
For example, if you need to test a service available only in a specific country, you can use proxies to make it appear as if your requests originate from that location.
Anonymize requests
Proxies enable you to anonymize your requests by masking your real IP address and replacing it with the proxy server's IP. This is especially useful when performing sensitive tasks such as competitive research, accessing protected resources, or avoiding tracking mechanisms on websites.
In addition to hiding your identity, proxies also add a layer of security by isolating the client's network from potentially malicious servers. For instance, if you need to access untrusted endpoints or perform penetration tests, you can route traffic through a proxy to ensure your actual network remains protected.
Avoid IP bans during web scraping or API testing
Web scraping and API testing often require sending a large number of requests in a short period, which can trigger rate limits or IP bans. By integrating rotating proxies with cURL, developers can distribute these requests across multiple IP addresses, effectively bypassing server-side restrictions and avoiding detection.
Using proxies also helps you to access resources during scraping or testing operations, as servers often flag repeated requests from a single IP address as suspicious. With cURL's scripting capabilities and proxy support, you can maintain consistent data retrieval or testing workflows without interruptions caused by bans or throttling.
Introducing proxies: types, cURL protocol support, and choosing the right one
When you use cURL with proxies, it’s important to understand the different types of proxies and their features. Each type serves unique use cases, and cURL's flexibility allows seamless integration with various proxy protocols.
Choosing the right proxy depends on the specific requirements of your task, such as anonymity, speed, or bypassing restrictions.
Types of proxies
- Residential proxies use IP addresses that ISPs assign to real devices (such as home computers or mobile phones). They are tied to physical locations, which makes them reliable and harder to detect. You can use them to bypass geographic restrictions, perform web scraping, or access region‑specific content. Keep in mind that residential proxies are usually more expensive because they are more authentic and less likely to be blocked.
- Datacenter proxies run from data centers and do not rely on an ISP. They offer high speed and scalability, making them suitable for tasks that require rapid request handling, such as API testing or bulk scraping. These proxies are cost-effective and widely available, making them a popular choice for developers. The downside of datacenter proxies is that they are more likely to be flagged or blocked by websites, as their traffic often appears automated. They are better suited for use cases where speed and volume are prioritized over stealth and location specificity.
- Mobile proxies use IP addresses that telecom providers assign to mobile devices. These proxies are useful for frequently rotating IPs as devices switch between networks. Mobile proxies are particularly helpful for tasks that require mimicking mobile user behavior, such as testing mobile applications or accessing mobile-only content. Due to their versatility and limited availability, mobile proxies are often more expensive. They are primarily used in scenarios where mobile-specific traffic simulation or high-level anonymity is required.
Protocols supported by cURL
- HTTP/HTTPS proxies: cURL supports HTTP and HTTPS proxies, which are the most commonly used types. These proxies route web traffic over the HTTP or HTTPS protocol, allowing developers to interact securely with web servers. HTTPS proxies, in particular, encrypt traffic, ensuring that sensitive data remains secure during transmission.
- SOCKS4 and SOCKS5 proxies: SOCKS proxies operate at a lower level compared to HTTP/HTTPS proxies, making them more versatile. SOCKS4 supports only TCP traffic, while SOCKS5 adds support for UDP traffic and authentication, allowing more flexible and secure connections. SOCKS proxies are often used for advanced use cases like peer-to-peer connections, torrenting, and bypassing firewalls.
How to choose the right proxy for cURL
Selecting the right proxy depends on your specific needs and goals. If your primary focus is stealth, avoiding IP bans, and accessing geo-restricted content, residential proxies are your best choice, whereas high-speed and high-volume tasks like API testing or bulk scraping are better suited by datacenter proxies.
If your application involves mobile-specific scenarios, such as testing mobile networks or simulating mobile user behavior, you can use mobile proxies to make your traffic appear as if it is coming from a real mobile device.
Additionally, ensure that the proxy type you choose supports the protocol and features required for your operation. cURL’s support for various proxy types and protocols ensures you have the flexibility to tailor your setup to meet your exact needs.
Setting up cURL with proxies
Using proxies with cURL lets you customize your request routing to improve privacy and location flexibility, and to bypass restrictions.
This section explains the syntax for configuring proxies in cURL, demonstrates common proxy setups, and provides a practical example of using an authenticated residential proxy.
Syntax overview for using proxies with curl
Use the following flags to configure proxies in cURL:
--proxy
for specifying the proxy server.--socks5
for using a SOCKS5 proxy.--proxy-user
for providing authentication credentials to the proxy server.
The basic syntax for setting up a proxy with cURL is as follows:
curl --proxy [proxy_url:port] [target_url]
This command sends your request to the target_url
through the specified proxy_url
and port. You can add more options for authentication or SOCKS support.
Examples for common proxy configurations
HTTP proxy
To route a request through an HTTP proxy, use the --proxy
flag followed by the proxy server address and port:
curl --proxy http://proxy.example.com:8080 https://api.example.com/data
In this example, cURL sends the request to https://api.example.com/data
via the HTTP proxy proxy.example.com
on port 8080
. This setup is ideal for basic proxy use cases.
SOCKS proxy
For SOCKS proxies, cURL provides the --socks5
flag. This is particularly useful for handling both TCP and UDP traffic:
curl --socks5 socks5://proxy.example.com:1080 https://api.example.com/data
The above command sends the request through the SOCKS5 proxy proxy.example.com
on port 1080
. If your proxy uses SOCKS4, you can replace --socks5
with --socks4
.
Authentication
When the proxy server requires authentication, the --proxy-user
flag can be used to provide credentials in the username:password
format:
curl --proxy http://proxy.example.com:8080 --proxy-user user123:password456 https://api.example.com/data
This ensures the proxy server authenticates the request before forwarding it to the target URL.
Real-world example: Sending a cURL request via an authenticated residential proxy
Here’s a practical example that combines multiple configurations. Imagine you want to access geo-restricted content via an authenticated residential proxy:
curl --proxy http://us.residential.proxy.com:3128 \
--proxy-user user123:securepass \
-H "User-Agent: Mozilla/5.0" \
https://restricted-website.com/resource
--proxy
specifies the residential proxy serverus.residential.proxy.com
on port3128
.--proxy-user
provides the username (user123
) and password (securepass
) for authentication.-H
sets a customUser-Agent
header to simulate a browser request, which can help bypass detection mechanisms.
This configuration is suitable for tasks such as scraping data from region‑specific websites or testing applications in different locations while ensuring the connection remains secure and authenticated.
Advanced techniques for using cURL with proxies
This section explores methods for rotating proxies, using proxy lists, managing request automation, handling failures, and ensuring secure proxy connections.
Rotating proxies with cURL for web scraping
Rotating proxies help you avoid IP bans during high‑volume web scraping. They change the IP address for each request so that your requests come from different addresses.
Step-by-step example of rotating proxies with cURL
1. Prepare a list of proxies: Create a text file containing multiple proxy addresses, one per line, e.g., proxies.txt
.
http://proxy1.example.com:8080
http://proxy2.example.com:8080
http://proxy3.example.com:8080
2. Write a script for rotation: Use a shell script or a programming language like Python to read proxies from the file and cycle through them for each request.
while read proxy; do
curl --proxy "$proxy" https://example.com/data
done < proxies.txt
This approach ensures each request is sent via a different proxy, reducing the risk of detection or bans.
Using cURL with proxy lists
Automating request rotation
Automating requests with a proxy list enables you to scrape data or test APIs across multiple endpoints efficiently. Combine cURL with tools like xargs
to parallelize requests and maximize throughput.
cat proxies.txt | xargs -I {} -P 5 curl --proxy {} https://example.com/data
-P 5
allows up to 5 parallel requests at a time.-I {}
replaces{}
with each proxy address from the list.
This method is particularly useful for large-scale scraping tasks, where speed and rotation are critical.
Handling failures gracefully
When a proxy fails to connect or the server blocks a request, handle these scenarios gracefully by implementing retries or skipping to the next proxy.
for proxy in $(cat proxies.txt); do
curl --proxy "$proxy" --retry 3 --retry-delay 5 https://example.com/data \
|| echo "Failed with $proxy"
done
--retry 3
retries the request up to three times.--retry-delay 5
waits 5 seconds between retries.
This ensures your workflow continues even when some proxies fail.
Using proxies securely with HTTPS and TLS
When handling sensitive data or accessing secure endpoints, ensure that your proxies support HTTPS and TLS encryption. This helps to maintain data privacy. cURL allows you to enforce secure connections and verify certificates during proxy usage.
Enforcing secure connections
To use an HTTPS proxy, specify the proxy URL with the https://
prefix:
curl --proxy https://secure-proxy.example.com:443 https://example.com/data
This ensures traffic between your client and the proxy is encrypted.
Verifying certificates
By default, cURL verifies the proxy's SSL/TLS certificate. To disable this (not recommended), use the --proxy-insecure
flag. For added security, provide a custom CA certificate bundle for validation:
curl --proxy https://secure-proxy.example.com:443 \
--cacert /path/to/ca-bundle.crt \
https://example.com/data
This setup is ideal for environments where strict security compliance is required, such as financial applications or sensitive data transfers.
By combining these advanced techniques, you can build robust workflows that maximize efficiency, maintain security, and reduce the risk of detection during automated tasks.
Common issues and how to fix them
When using cURL with proxies, you may encounter errors or connection problems that interrupt your workflows. Understanding how to diagnose and resolve these issues is essential for smooth operations. This section outlines common problems like proxy authentication errors, timeouts, and connection failures, along with debugging tips for identifying and addressing the root causes.
Diagnosing cURL errors with proxies
Error 407: proxy authentication required
This error occurs when the proxy server expects authentication credentials but none are provided or the credentials are incorrect.
Steps to fix:
1. Provide authentication credentials: Use the --proxy-user
flag with the username:password
format:
curl --proxy http://proxy.example.com:8080 --proxy-user user123:pass123 https://example.com/data
2. Check your credentials: Ensure the username and password are correct. If possible, test them directly with another proxy-compatible tool.
3. Confirm proxy settings: Verify that the proxy supports authentication and that the URL and port are correct.
Timeout issues
Timeout errors occur when cURL fails to receive a response from the proxy or the target server within a set time. This can happen due to network instability, overloaded proxies, or restrictive firewalls.
Steps to Fix:
1. Increase timeout settings: Use the --max-time
flag to extend the maximum time allowed for the operation:
curl --proxy http://proxy.example.com:8080 --max-time 60 https://example.com/data
2. Test proxy performance: Check if the proxy is slow or unresponsive by testing it with a lightweight request or a different tool.
3. Switch proxies: If the proxy remains unresponsive, try a different proxy server to determine if the issue is specific to the proxy.
Connection failures
Connection failures can occur when the proxy server is unreachable, the port is blocked, or there’s an issue with DNS resolution.
Steps to Fix:
1. Check proxy availability: Ping the proxy server or use telnet
to ensure the server is reachable on the specified port:
telnet proxy.example.com 8080
2. Verify network configuration: Ensure your local network settings, such as firewalls or VPNs, are not blocking proxy traffic.
3. Specify DNS resolution: If DNS resolution is failing, use the --resolve
flag to manually map the domain to an IP address:
curl --resolve proxy.example.com:8080:192.168.1.1 \
--proxy http://proxy.example.com:8080 \
https://example.com/data
Tips for debugging
1. Using -v
or --trace
for verbose output
cURL provides detailed logs that can help identify the root cause of proxy-related issues. Add the -v
flag to your cURL command to display connection details:
curl -v --proxy http://proxy.example.com:8080 https://example.com/data
Look for information like proxy authentication attempts, timeouts, or DNS resolution failures in the output.
2. Use trace logs for deep debugging: For even more detailed output, use the --trace
option to log the entire process, including headers and data:
curl --trace trace.log --proxy http://proxy.example.com:8080 https://example.com/data
Review the log file to identify where the request failed.
Checking network settings and proxy logs
If verbose logs don’t provide enough clarity, check your network and proxy logs for additional insights:
- Inspect network configuration: Ensure that your local network permits outbound traffic to the proxy server.
- Review proxy server logs: If you have access to the proxy server, review its logs to see if your requests are reaching the server and why they might be failing.
- Run a network diagnostic tool: Use tools like
traceroute
or cURL alternatives (e.g.,wget
) to test connectivity and rule out external factors.
By following these diagnostic steps and debugging tips, you can quickly identify and resolve most issues encountered when using cURL with proxies, ensuring smoother and more reliable operation.
Advanced tips for optimizing cURL with proxies
Optimizing cURL with proxies involves following best practices to enhance performance, minimize errors, and maximize efficiency. This section covers techniques like using proxy managers, handling rate limits, avoiding common pitfalls, and testing proxy setups to ensure your configuration works effectively.
Best practices for performance
Combining cURL with proxy managers
Using proxy managers can streamline and optimize your workflow, especially for large-scale tasks like web scraping or automated testing. Proxy managers simplify tasks like rotating proxies, handling retries, and managing proxy pools.
1. Use proxy management tools: Tools like ProxyMesh, Bright Data, or open-source solutions (e.g., RotatingProxies) can help automate proxy rotation and ensure reliable connections.
Example: Instead of manually rotating proxies in your script, a proxy manager can dynamically assign proxies to your requests.
2. Integrate with cURL: Configure your proxy manager as the default proxy for cURL. For instance:
curl --proxy http://proxy-manager.example.com:8000 https://example.com/data
If you exceed the rate limits set by servers, you may trigger an IP ban, or the server might artificially slow down your traffic (throttling). Proper rate management ensures you can scrape smoothly without running into any anti-scraping mechanisms.
Introduce delays between requests: Use sleep
commands in shell scripts or delay functions in programming languages to space out requests. Example in Bash:
for proxy in $(cat proxies.txt); do
curl --proxy "$proxy" https://example.com/data
sleep 2
done
- Monitor HTTP status codes: Pay attention to status codes like
429 Too Many Requests
. When detected, introduce a longer delay before resuming requests. - Leverage proxy pools: Distribute requests across multiple proxies to avoid hitting individual proxy rate limits.
Avoiding common pitfalls
Incorrect proxy format
Using an incorrect proxy format can lead to connection errors. Always verify the syntax and make sure that the proxy URL includes the protocol hostname,and port.
- Correct format:
http://proxy.example.com:8080
- Common error: Omitting the protocol (
proxy.example.com:8080
)
Unsupported protocols
You should make sure that the proxy server supports the protocol you want to use with your request (e.g., HTTP, HTTPS, SOCKS).
For HTTP/HTTPS:
curl --proxy http://proxy.example.com:8080 https://example.com/data
For SOCKS proxies:
curl --socks5 proxy.example.com:1080 https://example.com/data
Credential mismatches
When using authenticated proxies, double-check the credentials provided. Even a small typo in the username or password can result in errors.
How to test your proxy setup effectively
Using IP check services
One of the simplest ways to confirm that your proxy setup is working is by using an IP check service that returns your current IP address, allowing you to verify that the proxy is active.
Testing with cURL:
Without a proxy:
curl https://api.ipify.org
Output: Your real IP address.
With a proxy:
curl --proxy http://proxy.example.com:8080 https://api.ipify.org
Output: The proxy server’s IP address.
Simulating real use cases
Test your proxy setup in realistic scenarios to ensure it handles the intended workload. For example:
- Test proxy rotation by making multiple requests and verifying different IPs.
- Simulate slow networks or high traffic loads to observe proxy performance.
Using debugging tools<
Leverage cURL’s -v
or --trace
flags to debug your proxy configuration and ensure that requests are routed correctly.
curl -v --proxy http://proxy.example.com:8080 https://example.com/data
By following these pro tips, you can optimize your cURL workflows with proxies, ensuring performance, reliability, and compliance with server restrictions.
Use cases for cURL with proxies
Using cURL with proxies lets you perform tasks efficiently, maintain privacy, bypass restrictions, and automate workflows. Below are some common use cases.
Web scraping with rotating proxies
Web scraping often requires accessing a large number of web pages in a short period of time. Many websites implement anti-bot mechanisms such as IP blocking or rate limiting. By using cURL with rotating proxies, you can simulate requests from different users, effectively bypassing these restrictions.
Example:
- Rotating proxies: Automating web scraping by rotating between several proxy IPs. This ensures each request is made from a different address, reducing the chances of being blocked.
- Maximizing data collection: By using cURL with a proxy manager or a proxy pool, you can rotate through hundreds of proxies to gather data from various websites without running into IP bans or throttling.
This technique is particularly useful for gathering large datasets or performing competitive intelligence without risking detection.
Testing website performance from different locations
Proxies allow you to test the performance and response times of a website from different geographic locations. This is especially useful for understanding latency and regional performance variations.
Example:
curl --proxy http://proxy-eu.example.com https://example.com/data
curl --proxy http://proxy-asia.example.com https://example.com/data
Regional performance comparison: Analysing website load times or uptime across various locations allows businesses to optimize content delivery, adjust server locations, and improve user experience.
This method helps ensure that websites perform well for users regardless of their physical location.
API monitoring and debugging with anonymity
When testing or debugging APIs, it’s often important to keep the source of the requests anonymous, especially in cases involving security audits or competitive research. Using cURL with proxies helps mask the requester’s identity while still capturing all necessary data.
When debugging APIs, for example, requests can be sent through proxies to avoid revealing the original IP address. This can help prevent tracking, rate limiting, or unwanted restrictions.
For sensitive APIs, such as financial or private services, ensuring anonymity can prevent unauthorized access and maintain confidentiality during debugging or testing.
This use case is especially valuable when running automated API tests or conducting penetration testing without exposing internal systems.
These real-world applications demonstrate how combining cURL with proxies can enhance your ability to scrape data, test websites, and monitor APIs while maintaining flexibility, anonymity, and control over your network behavior.
This article explained how to use cURL
with proxies. You learned how proxies help you bypass restrictions, hide your IP address, and support efficient web scraping and testing.
If you’re looking for reliable proxy services that work with cURL
, explore our range of proxy options for easy integration and optimized performance.
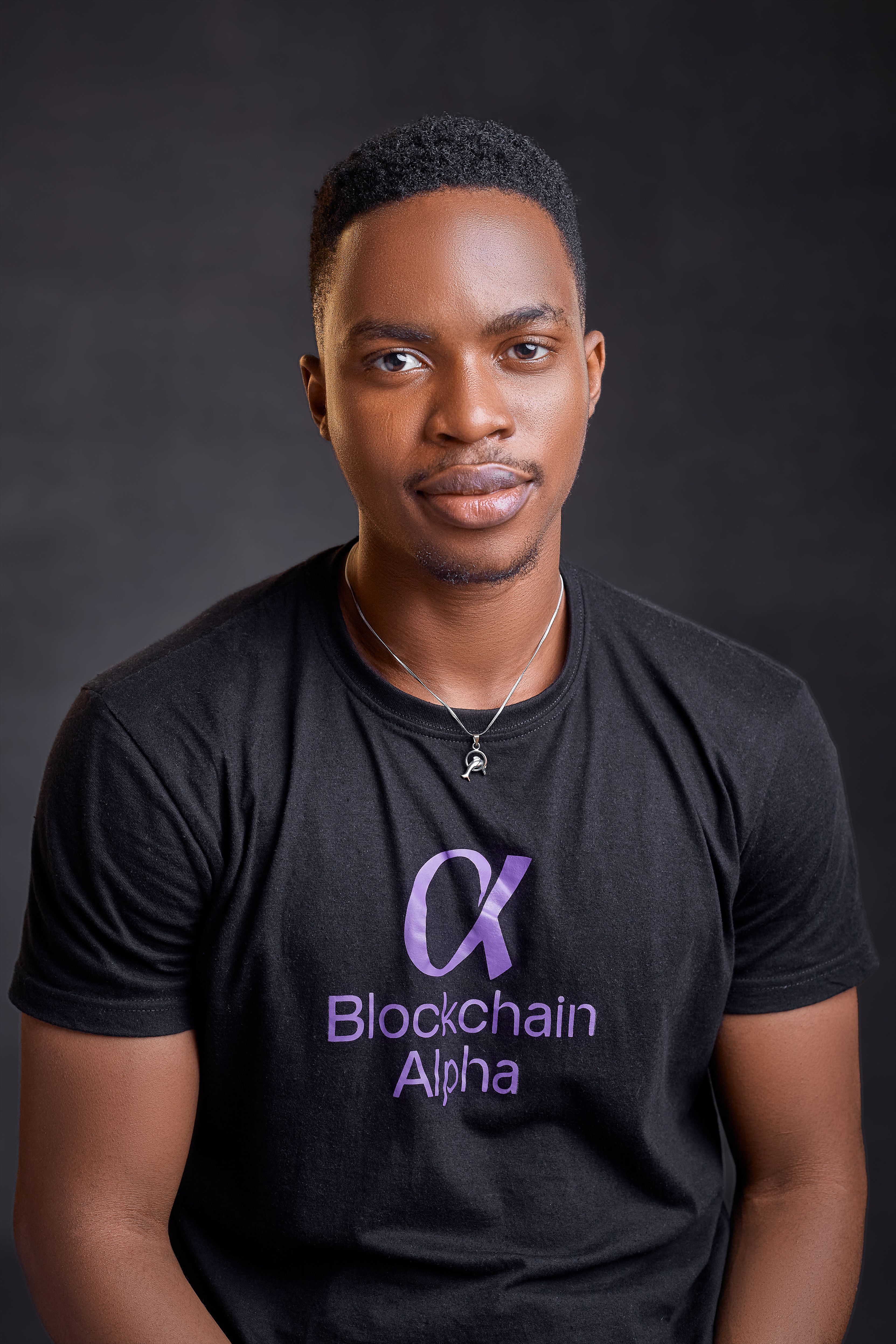
John Fáwọlé
John Fáwọlé is a technical writer and developer. He currently works as a freelance content marketer and consultant for tech startups.
Related posts
When downloading files or scraping data from the web, you might find it helpful to route your traffic through a proxy. Using a proxy with wget offers lots of advantages, like bypassing...
When you navigate the internet, your device doesn’t always connect directly to the destination server. Instead, requests are often routed through a proxy before reaching their destinat...
Tracking an IP address can help you gather information about the physical location of a device that’s connected to the internet. It can also reveal some technical and contextual inform...